Application testing example
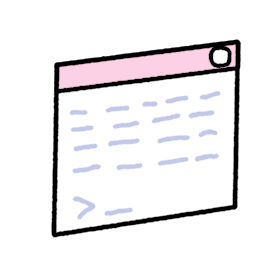
The package provides unit testing functionality through an instance of the
Testing can be performed both on the server side and on the browser side. So, it is also possible to run tests in the browser from the NODEJS application.
Consider an example of testing an application.
First, let's create a project and install the fcf-framework-unitest package. After that, we will add the main index.js file, which will export a function that will always return the value
$ mkdir application
$ cd application
$ npm init
$ npm install --save fcf-framework-unitest
index.js file:
module.exports = function() {
return 1;
}
Test declaration
Now let's add a unit test to test our function. To do this, create a file tests/localTests.js and declare a test using the function
tests/localTests.js file:
const someFunction = require("../index.js");
fcf.test("Local test", (a_unitest )=>{
a_unitest .equal (someFunction(), 1);
});
To declare and connect a test, the
The function
Function signature: [simple or async] void a_cbtest(
Running tests on the server side
Now you need to run the test, for this you need to execute the
run-tests.js file:
let unitest = require("fcf-framework-unitest");
unitest .run ({
include: [":tests/localTests.js"],
})
.finally (()=>{
process.exit(0);
});
And let's run the test
$ node run-tests.js
2023-05-13 03:26:51.018 [PID:228207] [LOG] [MOD:UniTest]: ====================================================
2023-05-13 03:26:51.023 [PID:228207] [LOG] [MOD:UniTest]: Start testing on the server side...
2023-05-13 03:26:51.023 [PID:228207] [LOG] [MOD:UniTest]: Parts: *
2023-05-13 03:26:51.024 [PID:228207] [LOG] [MOD:UniTest]: Groups: *
2023-05-13 03:26:51.024 [PID:228207] [LOG] [MOD:UniTest]: Tests: *
2023-05-13 03:26:51.025 [PID:228207] [LOG] [MOD:UniTest]:
2023-05-13 03:26:51.025 [PID:228207] [LOG] [MOD:UniTest]: Start local tests...
2023-05-13 03:26:51.025 [PID:228207] [LOG] [MOD:UniTest]: --------------------
2023-05-13 03:26:51.026 [PID:228207] [LOG] [MOD:UniTest]: Test [default][default][Local test] running ...
2023-05-13 03:26:51.027 [PID:228207] [LOG] [MOD:UniTest]: Test [default][default][Local test] is completed.
2023-05-13 03:26:51.028 [PID:228207] [LOG] [MOD:UniTest]:
2023-05-13 03:26:51.028 [PID:228207] [LOG] [MOD:UniTest]: ----------------------------------------------------
2023-05-13 03:26:51.029 [PID:228207] [LOG] [MOD:UniTest]: 1 test have been completed.
2023-05-13 03:26:51.029 [PID:228207] [LOG] [MOD:UniTest]: Errors: 0; Successfully: 1; Total: 1
Running tests on the browser side
Unit testing of the framework can also be done on the browser side, with the ability to run from a NODEJS script.
First, let's create a simple one-page application. Create a NODEJS server to serve the index.html page to the browser on port 8080
server.js file:
let libExpress = require("express");
let libPath = require("path");
let server = libExpress();
server.use("/", libExpress.static(__dirname));
server.get("/", libExpress.static(libPath.join(__dirname, "index.html")));
const port = 8080;
server.listen(port, () => {
console.log(`Start listing on ${port} port`);
});
And let's make a simple HTML page, which will contain an input field, when filled in, the data will be duplicated in a div with an index label
The fcf-framework-core:fcf.min.js framework file must be included in the page header in order to be able to use the unit testing functionality.
index.html file:
<html>
<head>
<script src="/node_modules/fcf-framework-core/fcf.min.js"></script>
</head>
<body>
<fieldset>
<p>
<input id="input">
</p>
<p>
<div id="label" style="min-height: 1.2em;"></div>
</p>
</fieldset>
<script>
document.getElementById("input").addEventListener("input", (a_event)=>{
document.getElementById("label").innerHTML = a_event.target.value;
})
</script>
</body>
</html>
Let's run our test application:
$ node server.js
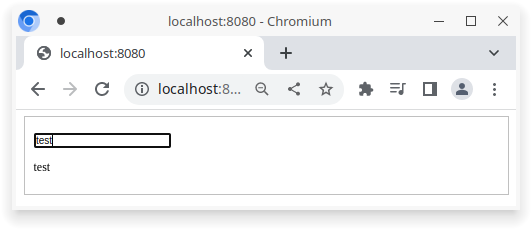
Now let's create a test function for our page in the file tests/browserTests.js
tests/browserTests.js file:
fcf.test("Browser test", (a_unitest )=>{
const input = document.getElementById("input");
const label = document.getElementById("label");
input.value = "test";
input.dispatchEvent(new Event("input"));
a_unitest .equal (label.innerHTML, input.value);
});
It remains only to change the code for calling the
webProcesses option
The
Description of the elements of the webProcesses array:
-
string |[string ] command - Run command, which can be given as a single string or an array of command line arguments. -
integer startTimeout =1000 - Time in milliseconds to wait for the application to start. The executable script transfers control to the following commands only after the specified delay has elapsed.
webTestingPages option
In addition, you need to tell the
[
Object properties:
-
string url - URL of the page being tested -
[
string ] include = [] - An array of additionally included URLs to JS files on the browser side, where tests can be located. -
integer |string wait =0 - Waiting time before testing, after page load. If the parameter is a string, then it must contain the path to a global asynchronous function that will return control after all page components have been loaded.
webBrowsers option
The choice of the browser to be launched is determined by the webBrowsers parameter. This must be an array, where the element specifies for which browser the test will be performed. If the parameter has the value [
The value of the array element is the name of the command to be executed, so the path to the specified browser must be specified in the PATH environment variable. This is especially important on Windows!
If the array element is an array, then the test will run against the first browser in the nested array that runs successfully.
By default, the webBrowsers option is set to [[
And so, the run-tests.js file should take the following form:
let unitest = require("fcf-framework-unitest");
unitest .run ({
include: [":tests/localTests.js"],
webProcesses: [{
command: "node ./server.js",
}],
webTestingPages: [
{
url: "http://localhost:8080",
include: ["tests/browserTests.js"]
}
]
})
.finally (()=>{
process.exit(0);
});
Let's start testing
$ node run-tests.js
2023-05-13 05:15:42.144 [PID:230049] [LOG] [MOD:UniTest]: ====================================================
2023-05-13 05:15:42.148 [PID:230049] [LOG] [MOD:UniTest]: Start testing on the server side...
2023-05-13 05:15:42.149 [PID:230049] [LOG] [MOD:UniTest]: Parts: *
2023-05-13 05:15:42.149 [PID:230049] [LOG] [MOD:UniTest]: Groups: *
2023-05-13 05:15:42.150 [PID:230049] [LOG] [MOD:UniTest]: Tests: *
2023-05-13 05:15:42.150 [PID:230049] [LOG] [MOD:UniTest]:
2023-05-13 05:15:42.151 [PID:230049] [LOG] [MOD:UniTest]: Start local tests...
2023-05-13 05:15:42.151 [PID:230049] [LOG] [MOD:UniTest]: --------------------
2023-05-13 05:15:42.151 [PID:230049] [LOG] [MOD:UniTest]: Test [default][default][Local test] running ...
2023-05-13 05:15:42.153 [PID:230049] [LOG] [MOD:UniTest]: Test [default][default][Local test] is completed.
2023-05-13 05:15:42.231 [PID:230049] [LOG] [MOD:UniTest]:
2023-05-13 05:15:42.231 [PID:230049] [LOG] [MOD:UniTest]: Start web tests...
2023-05-13 05:15:42.232 [PID:230049] [LOG] [MOD:UniTest]: --------------------
2023-05-13 05:15:42.232 [PID:230049] [LOG] [MOD:UniTest]: Start process: node ./server.js
2023-05-13 05:15:42.237 [PID:230049] [LOG] [MOD:UniTest]: Child process "node" started with pid 230056
2023-05-13 05:15:42.374 [PID:230049] [LOG] [MOD:UniTest]: Sub process "node" stdout:
[node PID: 230056]> Start listing on 8080 port
[node PID: 230056]>
2023-05-13 05:15:43.238 [PID:230049] [LOG] [MOD:UniTest]: Start back server on port 4589
2023-05-13 05:15:43.245 [PID:230049] [LOG] [MOD:UniTest]: Start browser 'google-chrome "http://localhost:8080?___fcf_unitest&___fcf_unitest_host=localhost&___fcf_unitest_port=4589&___fcf_unitest_tests=undefined&___fcf_unitest_groups=undefined&___fcf_unitest_parts=undefined&___fcf_unitest_id=d54c8793ad107a8ac4e2bd4c3fac5ac8&___fcf_unitest_include=%5B%22tests%2FbrowserTests.js%22%5D"' ...
2023-05-13 05:15:47.330 [PID:230049] [LOG] [MOD:UniTest; BROWSER:google-chrome]: Test [default][default][Browser test] running ...
2023-05-13 05:15:47.336 [PID:230049] [LOG] [MOD:UniTest; BROWSER:google-chrome]: Test [default][default][Browser test] is completed.
2023-05-13 05:15:47.360 [PID:230049] [LOG] [MOD:UniTest]:
2023-05-13 05:15:47.360 [PID:230049] [LOG] [MOD:UniTest]: ----------------------------------------------------
2023-05-13 05:15:47.361 [PID:230049] [LOG] [MOD:UniTest]: 2 tests have been completed.
2023-05-13 05:15:47.361 [PID:230049] [LOG] [MOD:UniTest]: Errors: 0; Successfully: 2; Total: 2
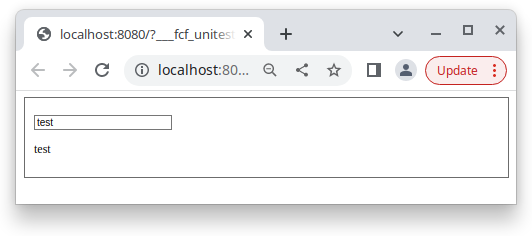